As a web developer, the responsiveness and interactivity of your web application are key components of the user experience. To enhance the user experience of your application, you’ll need to add dynamic behaviors and have control over the time when certain pieces of code in your application are executed.
The method setTimeout() is an excellent tool for this, as it allows you to schedule the execution of code, orchestrate animations, create timers, and manage asynchronous operations in your application.
Therefore, it is important to understand setTimeout()’s syntax, how to use it, and how you can apply it in your next project. This article will explore just that and equip you with the right knowledge to allow you to make the most of the setTimeout() method.
JavaScript’s setTimeout Function
setTimeout() is a global method bound to the window object and it is used to execute a function or a piece of code, after a certain period. For example, if you have a function that you want to execute after four seconds, setTimeout() is the method you’d use to achieve this.
When used, the setTimeout() method sets a timer that counts down until the time you specify elapses, then executes the function or code you’ve passed into it.

Being a global method means that setTimeout() is found within the global object and thus is available everywhere without the need for importation. The global object, which is provided by the Document Object Model(DOM) in the browser, is referenced by the property name window.
Therefore, we can use window.setTimeout() or simply setTimeout() as the global object window will be implied. window.setTimeout() and setTimeout() have no difference.
The general syntax for setTimeout() is as follows:
setTimeout(function, delay)
- function – this is the function to be executed after a given time.
- delay – time in milliseconds after which the function passed is to be executed. 1 second is equivalent to 1000 milliseconds.
When using setTimeout() the delay you specify should be a numerical value to avoid unwanted outcomes. The delay argument is optional. If it is not specified, it defaults to 0 and the function passed is executed immediately.
setTimeout() returns a unique identifier known as a timeoutID, which is a positive integer that uniquely identifies the timer created when a setTimeout() method is called.
It is also important to note that setTimeout() is asynchronous. Its timer does not stop the execution of other functions in the call stack.
How To Use setTimeout()
Let us look at examples showing how to use the setTimeout() method:
// setTimeout() with a function declaration
setTimeout(function () {
console.log("Hello World after 3 seconds");
}, 3000)
// setTimeout() with an arrow function
setTimeout(() => {
console.log("Hello World in an arrow function - 1 second")
}, 1000)
Output:
Hello World in an arrow function - 1 second
Hello World after 3 seconds

In the output above, the second setTimeout() logs out its output first because it has a shorter delay of 1 second, compared to the first one which has a delay of 3 seconds. As noted earlier, setTimeout() is asynchronous. When the first setTimeout() with a delay of 3 seconds is called, a 3- seconds timer is started but it does not stop the execution of the other code in the program.
As the method counts down from 3, the rest of the code in the call stack is executed. In this example, the next piece of code is the second setTimeout() with a delay of 1 second. Since it has a much shorter delay, this is why its code is executed before the first setTimeout()
When using setTimeout(), you don’t have to directly write the function in the setTimeout() method.
// function declaration
function greet() {
console.log("Hello there!")
}
// store a function in a variable - function expression
const speak = function () {
console.log("How are you doing?")
}
// using an arrow function
const signOff = () => {
console.log("Yours Sincerely: Geekflare:)")
}
// pass in a function reference to setTimeout()
setTimeout(greet, 1000)
setTimeout(speak, 2000)
setTimeout(signOff, 3000)
Output:
Hello there!
How are you doing?
Yours Sincerely: Geekflare:)
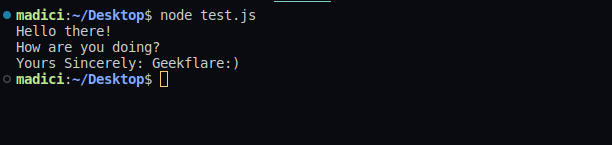
When we define a method elsewhere, then pass it setTimeout(), what we pass into the setTimeout() method is a reference to the function we want to execute after a given time.
setTimeout() With Additional Parameters
setTimeout() has an alternative syntax that allows you to pass in additional parameters into the setTimeout() method. These parameters are going to be used in the function you execute after the delay.
The syntax is as follows:
setTimeout(functionRef, delay, param1, param2, /* …, */ paramN)
You can pass in any given number of additional parameters depending on the number of arguments needed by the function you reference.
Consider the function below:
function greet (name, message) {
console.log(`Hello ${name}, ${message}`)
}
If you want to execute the above function after a certain amount of time using setTimeout(), you can do it as shown below:
// setTimeOut() with additional parameters
function greet (name, message) {
console.log(`Hello ${name}, ${message}`)
}
setTimeout(greet, 2000, "John", "happy coding!");
Output:
Hello John, happy coding!

Remember, if we define a function elsewhere and then pass it into setTimeout(), what we pass in is simply a reference to the function. In the example above, we pass in the reference greet and not greet(), which calls the function. We want setTimeout() to be the one calling the function using its reference.
This is why we cannot directly pass in the additional parameters, as shown below:
// This results in an ERR_INVALID_ARG_TYPE error
setTimeout(greet("John", "happy coding!"), 2000);
In the code above, the greet function is executed immediately without waiting for 2 seconds to elapse. An error is then thrown. The result of executing the code is shown below:

Cancelling setTimeout()
We can prevent the execution of a function scheduled using setTimeout() by using the method clearTimeout(). Such a thing can be necessary if we’ve set a function to execute after a certain period, but we don’t want the function to execute after certain conditions have been met or conditions change.
The syntax for clearTimeout() method is as shown below:
clearTimeout(timeoutID)
clearTimeout() takes a single argument, timeoutID, which is the unique identifier returned by the setTimeout() method.
Consider the example below:
function text() {
console.log("This is not going to be printed")
}
function greet() {
console.log("Hello after 5 seconds")
}
// Schedule the function text() to be executed after 3 seconds
const timeOutID = setTimeout(text, 3000);
// cancelt text() timeout time using clearTimeout()
clearTimeout(timeOutID)
console.log(`${timeOutID} cleared out`)
// Schedule the function greet() to be executed after 5 seconds
setTimeout(greet, 5000)
Output:
2 cleared out
Hello after 5 seconds

The function text() is not executed as clearTimeout() is used to cancel its timeout, thus preventing its execution.
Advantages of Using setTimeout()

Some of the advantages of using the setTimeout() method include:
- Delaying Code Execution – the primary function of setTimeout() is to enable developers to delay the execution of code. This is a crucial feature when creating animations, managing timed events, and also controlling the flow of asynchronous code. setTimeout() also frees up the main thread to run other code.
- Timer Implementation – setTimeout() provides a simple way to implement simple timers in an application without needing to use external libraries or perform complex date operations.
- Throttling and Debouncing – setTimeout() can be used to limit the number of times certain functions are called or actions performed, particularly during events such as scrolling or typing. With debouncing, your application waits for a certain period of time before calling a function. Throttling limits the number of function calls made within a given time period. setTimeout() can be used to achieve both of these
- Enhance User Experience – setTimeout() allows you to enhance the user experience of your application by controlling when certain actions happen, such as when notifications, alerts, pop-up messages, and animations are displayed. This is handy in preventing information overload on users, thus enhancing the user experience.
- Improve Web Performance – setTimeout() can be used to improve the performance and overall responsiveness of web applications by breaking down complex problems into smaller much simpler problems. These smaller problems can be handled inside a setTimeout(), allowing other parts of code to continue execution, thus not affecting the performance or responsiveness of an application.
Clearly, setTimeout() is both powerful and very useful when building applications using JavaScript.
Disadvantages of Using setTimeout()

Some of the disadvantages of using setTimeout() include:
- Inaccurate timing – setTimeout() cannot guarantee the exact time that a function will be called or an operation performed. At times other poorly written code lead to race conditions which will affect the operation of setTimeout(). When you use multiple overlapping setTimeout()s, you can’t always be sure of the order of execution, particularly if other asynchronous operations are involved
- Callback Hell – If you have too many nested setTimeout() calls, your code can become hard to read and debug. It will also be very difficult to follow the flow of logic in your application. Using too many setTimeout() can also lead to memory issues in your application if the setTimeout() calls are not handled properly.
Whereas setTimeout() can prevent some challenges when using it, by sticking to best practicing and good coding practices, you can minimize or completely avoid the disadvantages in your application.
Conclusion
The setTimeout() method can be used to delay the execution of functions. setTimeout is commonly used for animations, delayed loading of content, and handling timeouts for requests.
For instance, you can use setTimeout() to display alerts on your web pages. Whereas setTimeout() does not guarantee the exact time a function is executed, it guarantees its execution after a set delay.
You may also explore JavaScript ORM platforms for efficient coding.
-
Collins Kariuki is a software developer and technical writer for Geekflare. He has over four years experience in software development, a background in Computer Science and has also written for Argot, Daily Nation and the Business Daily Newspaper.
-
Narendra Mohan Mittal is a Senior Digital Branding Strategist and Content Editor with over 12 years of versatile experience. He holds an M-Tech (Gold Medalist) and B-Tech (Gold Medalist) in Computer Science & Engineering.
… read more