As a programmer, you’re bound to encounter errors while developing software. This might range from errors in your logic leading to unexpected results, errors arising from violating the rules of a programming language, to even errors that arise while running your program, among many others. These errors are commonly referred to as bugs.
Bugs are ubiquitous in every programming language regardless of how easy it is to learn or use the language.
In Python, for instance, although the language emphasizes readability, follows an expressive syntax, and is considered relatively easy to learn and use compared to other programming languages, you’re still not immune to programming errors when using Python.
Since errors are bound to arise, a good way to deal with them is to learn about the different types of errors that can occur and how they occur. This allows you to avoid or minimize these errors while programming and also know how to handle them when they arise.
Here are some of the Common Python errors that you might encounter while programming using the language:
SyntaxErrors
A Syntax error is an error that arises when you write code that violates the rules of the programming language you’re using. This results in an invalid line of code.
In Python, for instance, when printing out a string, it needs to be put in between quotation marks. Failure to do so results in a Syntax error.
A syntax error can also arise when you miss opening or closing parentheses, square brackets, or curly braces, misspell keywords or function names, miss colons at the end of flow control statements, or when you miss required operators in expressions.
Generally, syntax errors will arise if you violate a rule on how Python code should be written.
## syntax error arising from missing quotation mark
## around the string being printed
print("Hello World)
age = 20
## Syntax error arising from missing colon in an if statement
if age > 18
print("Age is over 18")
## Syntax error because '(' was never closed
def square(x:
return x * x
print(square(4))
Upon running the above code, you’ll run into an error message as shown below:
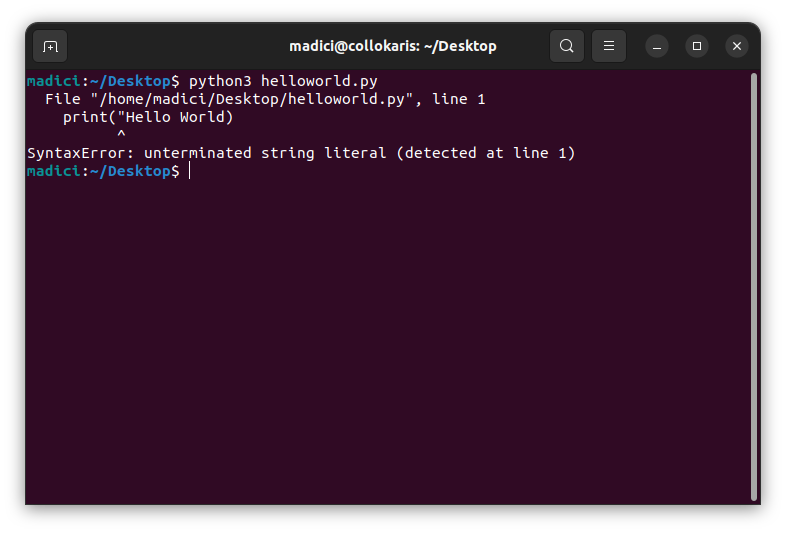
The error message from running the code is as follows:
File "/home/madici/Desktop/helloworld.py", line 1
print("Hello World)
^
SyntaxError: unterminated string literal (detected at line 1)
To solve these errors, use the right Python syntax as shown below:
print("Hello World")
age = 20
if age > 18:
print("Age is over 18")
def square(x):
return x * x
print(square(4))
IndentationError
Unlike other languages like Java, C, or C++, which use curly braces to separate blocks of code, Python uses indentation to define the hierarchy and structure of blocks of code. For instance, when writing control statements in Java, all the code to be executed once the condition has been evaluated is contained inside curly braces.
In Python, however, the block of code will be indented. A typical indentation in Python consists of four spaces or one tab. However, the number of spaces does not matter as long as it remains consistent throughout the code being written.
As a Python programmer, you’re likely to encounter indentation errors when you fail to add required indentation, such as when writing control statements or functions, when you use both tab and spaces to create indentations as it confuses the interpreter, when you put indentations in the wrong place or when your indentations are not consistent throughout your codebase.
An example of code that results in an indentation error is shown below:
age = 20
if age > 18:
print("Age is greater than 18")
print("You're allowed to drive")
else:
print("Age is less than 18")
The error messages resulting from the code above is shown below:

The error message from running the code is as follows:
File "/home/madici/Desktop/helloworld.py", line 3
print("Age is greater than 18")
^
IndentationError: expected an indented block after 'if' statement on line 2
To correct the errors indent the line after the if statement as an indentation is required and ensure it matches the indentation in the rest of the code as shown below:
age = 20
if age > 18:
print("Age is greater than 18")
print("You're allowed to drive")
else:
print("Age is less than 18")
TypeError
In Python, a TypeError is an exception that arises when you try to perform an operation using an incompatible data type. For instance, if you try to add a string and an integer or concatenate a string data type with an integer, you will encounter a TypeError.
You can also encounter TypeErrors when you use functions or methods with incorrect data types, when you try to use a noninteger index to access items in an iterable such as a list, or when you try to iterate through an object that cannot be iterable.
Generally, any operation using an incorrect data type will lead to a TypeError.
Examples of operations that can lead to TypeErrors are shown below:
# Type Error resulting from concatenating a string an an integer
age = 25
message = "I am " + age + " years old."
list1 = [1, "hello", 5, "world", 18, 2021]
#Type errors resulting from wrong usage of builtin in methods
print(sum(list1))
#TypeError resulting from adding a string and an integer
num1 = 10
num2 = "16"
print(num1 + num2)
#TypeError resulting from using a non integer index
list2 = ["hello", "from", "the", "other", "side"]
print(list2["1"])
The errors messages resulting from the code above shown is shown below:

A sample TypeError message from the code is shown below:
File "/home/madici/Desktop/helloworld.py", line 3, in <module>
message = "I am " + age + " years old."
~~~~~~~~^~~~~
TypeError: can only concatenate str (not "int") to str
To remove the errors, use the correct data types or type conversions as shown below:
age = 25
message = "I am " + str(age) + " years old."
list1 = [1, 5, 18, 2021]
print(sum(list1))
num1 = 10
num2 = "16"
print(num1 + int(num2))
list2 = ["hello", "from", "the", "other", "side"]
print(list2[1])
AttributeError
In Python, an AttributeError occurs when you try to use an attribute that does not exist on the object or call a method that does not exist on the object it is being called on. An AttributeError shows that an object does not have an attribute or method that is being called on it.
For instance, if you call a string method on an integer, you’ll run into an AttributeError because the method does not exist on the type of object you’re calling it on.
In the example shown below, the capitalize() method, which is used to convert the first letter of a string to uppercase, is being called on an integer. The result is an attribute error because int does not have the capitalize() method.
# AttributeError arising from calling capitalize() on an int value
num = 1445
cap = num.capitalize()
print(cap)
Running this code results in the error message shown below:

The AttributeError message from the code is as follows:
File "/home/madici/Desktop/helloworld.py", line 3, in <module>
cap = num.capitalize()
^^^^^^^^^^^^^^
AttributeError: 'int' object has no attribute 'capitalize'
To solve an AttributeError, ensure that the method or attribute you’re calling exists on the type of object you’re calling it on. In this case, calling capitalize() on a string data type solves this error as shown below:

ImportError
The ImportError in Python occurs when you try to import a module that cannot be found or is not accessible in your current environment. It could be that it is not yet installed, you have not have configured its path correctly or you’ve misspelled the module you’re trying to install.
An ImportError has a single child subclass called ModuleNotFoundError which is the error that is thrown when you try to import a module that cannot be found.
For instance, the code below with tries to import the data analysis library pandas throws such an error because the module is not yet installed.

The ImportError message generated is shown below:
File "/home/madici/Desktop/helloworld.py", line 1, in <module>
import pandas
ModuleNotFoundError: No module named 'pandas'
To solve such an error, ensure the modules you are trying to import have been installed. In case that does not solve the error, check whether you’re using the correct spelling for the module and the correct file path to access the module.
ValueError
This is an exception that occurs when a function in Python receives a value of the correct data type but the value is an inappropriate value. For instance the Math.sqrt() function used to find the square root of numerical values will return a ValueError if you pass in a negative number.
As much as the value will be of the correct type, that is a numerical value, being negative makes it an inappropriate value for the
The function int() which converts a number or a string will return a ValueError if you pass in a string that is not a numeric string value. Passing “123”, or “45” to the function returns no error as the strings can be converted to the appropriate integer value.
However, if you pass in a string that’s not a numeric string value such as “Hello” it returns a ValueError. This is because “Hello” although is a string, is inappropriate as it does not have an integer equivalent.
An example of code that generates a ValueError is shown below:
# Value error resulting from inappropriate int value in sqrt()
import math
num = -64
root = math.sqrt(num)
print(root)
# Value error resulting from passing a string with no integer
# equivalent into int() function
numString = "Hello"
num = int(numString)
print(num)
The errors from the code above is shown below:

The error message generated is as follows:
File "/home/madici/Desktop/helloworld.py", line 4, in <module>
root = math.sqrt(num)
^^^^^^^^^^^^^^
ValueError: math domain error
To correct the error, use appropriate values in the functions as shown below:
import math
num = 64
root = math.sqrt(num)
print(root)
numString = "5231"
num = int(numString)
print(num)
IOError
The IOError(Input/Output Error) is an exception that occurs when an input or output operation fails. This can be caused by trying to access a file that does not exist, insufficient disk storage in your device, trying to access a file that you don’t have sufficient permissions to access or when you try to access a file that is currently being used by other operations.
Methods such as open(), read(), write() and close() which are typically used when working with files are the ones likely to cause such an error.
Consider the code below which tries to open a file called “notes.txt” which does not exist. The code results in an IOError which raises the FileNotFoundError:

With the following error message:
File "/home/madici/Desktop/helloworld.py", line 2, in <module>
file1 = open("notes.txt", "r")
^^^^^^^^^^^^^^^^^^^^^^
FileNotFoundError: [Errno 2] No such file or directory: 'notes.txt'
To avoid the error above, all you need to do is ensure “notes.txt” file exists in the directory you running the terminal in. Another way to handle IOErrors is using the try except block, as shown below:
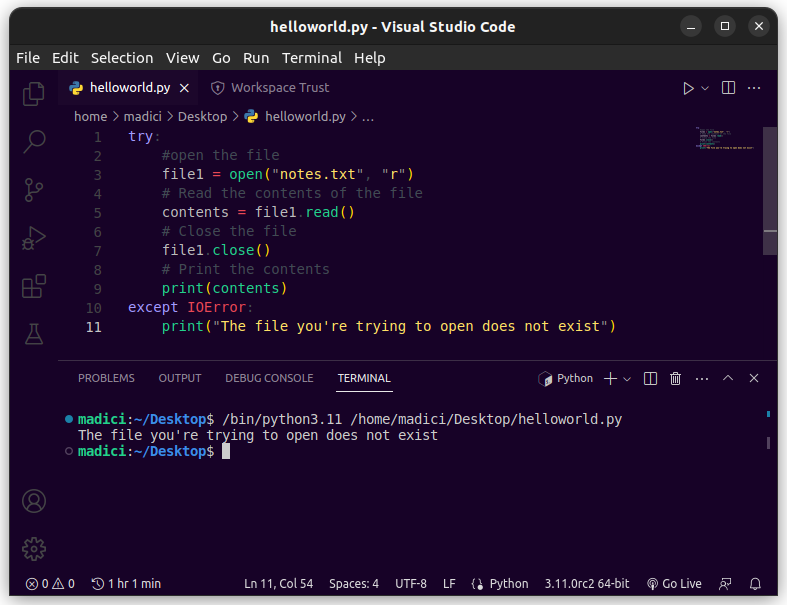
NameError
The NameError is an exception that you’ll encounter when you try to use a variable, function, or module that does not exist, is not defined in the current scope or has not been assigned a value.
Such an error typically occurs when you misspell variable or function names or use them before they are defined. Using a module without importing it will also result in a NameError.
The following code will result in a NameError exception:
# name error arises because the math module has not been imported
num = 64
root = math.sqrt(64)
print(root)
# NameError arises because x is used before it is defined
y = 23
print(x)
#NameEror because function name is not defined
def greet():
print("Good morning")
great() #ameError: name 'great' is not defined
The following error messages result from the code above:

A sample NameError message is shown below:
File "/home/madici/Desktop/helloworld.py", line 3, in <module>
root = math.sqrt(64)
^^^^
NameError: name 'math' is not defined
To solve such a NameError, ensure you’re not using modules before you import them, you’re not using variables or functions before you define them, and you’re not misspelling function or variable names:
import math
num = 64
root = math.sqrt(64)
print(root)
y = 23
print(y)
def greet():
print("Good morning")
greet()
IndexError
An IndexError is an exception that occurs when you try to access an index in a list or tuple that is out of range. Consider the list below:
list1 = [1, 2, 3, 4, 5]
The list has five elements. Python counts indexes from 0(zero). Therefore, the list above has indexes ranging from 0 to n-1, with n being the number or elements in the list. In this case, the index or the list will range from 0 to 4.
If you try to access an element at an index greater than 4, you’ll run into an IndexError because the index is out of range in the list you’re trying to access an element from. The code below generates an IndexError:
list1 = [1, 2, 3, 4, 5]
item = list1[6] #IndexError because the list index is out of range
print(item)
The error from the code is shown below:
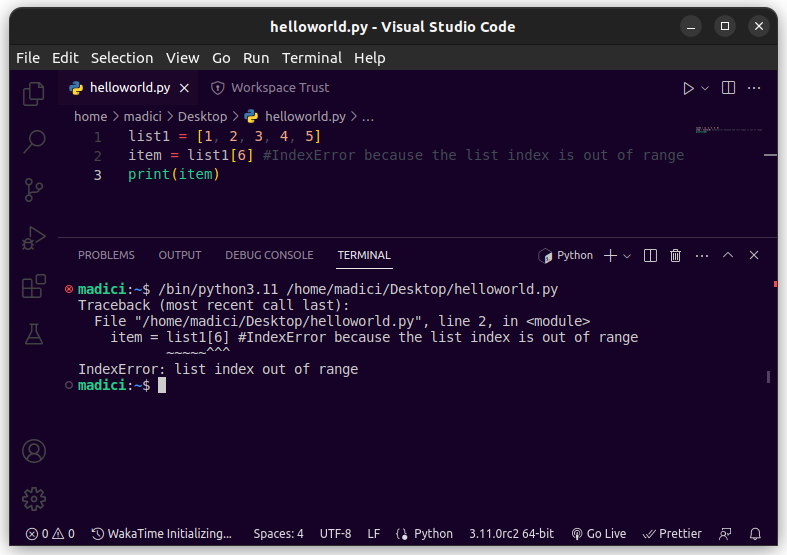
The IndexError message generated is as follows:
File "/home/madici/Desktop/helloworld.py", line 2, in <module>
item = list1[6] #IndexError because the list index is out of range
~~~~~^^^
IndexError: list index out of range
The best way to avoid an IndexError is to utilize the range() and len() functions to ensure you’re only accessing elements that are within range like so:
list1 = [1, 2, 3, 4, 5]
for i in range(len(list1)):
print(list1[i])
KeyError
A KeyError is an exception that occurs when you try to access an item from a dictionary using a key, and the key is not found in the dictionary. Consider the dictionary below:
cities = {"Canada": "Ottawa", "USA": "Washington", "Italy": "Rome"}
The keys in the dictionary are “Canada”, “USA”, “Italy”. You can access the items from the cities dictionary using the three keys. However, if you try to access an element using a key that does not exist, such as “Brazil”, you’ll run into a KeyError, as shown below:
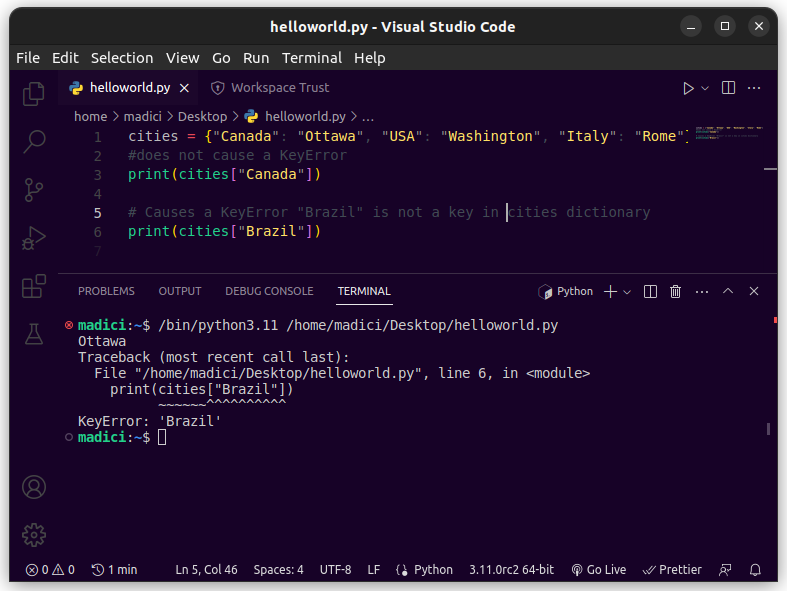
The KeyError message generated is shown below:
File "/home/madici/Desktop/helloworld.py", line 6, in <module>
print(cities["Brazil"])
~~~~~~^^^^^^^^^^
KeyError: 'Brazil'
To solve a KeyError, ensure the keys you are using to access elements in a dictionary are actually present in the dictionary. To do this, you can you an if…else statement like so:
cities = {"Canada": "Ottawa", "USA": "Washington", "Italy": "Rome"}
country = "Canada"
if country in cities:
print("The capital city of " + country + " is " + cities[country])
else:
print("The key " + country + " is not present in the cities dictionary")
This way, you’ll avoid running into KeyErrors when accessing elements from a dictionary
Conclusion
When coding in Python, regardless of your level of expertise, you’re bound to encounter errors. Therefore, make sure to familiarize yourself with the different types of errors highlighted in the article to ensure you’re able to handle them when they arise.
You may also explore some useful Python one-liners to simplify common tasks.